

If there’s an unhandled exception (or even a log message) in your JavaScript, you can click on it in the console and go straight to the source line, set a breakpoint and reload or re-trigger the code, and now you’ve got a fully interactive “stack trace” where you can inspect the values of local variables at all levels of the stack. I'm absolutely certain the number of times I've considered the behaviour a feature rather than an annoyance is 0.
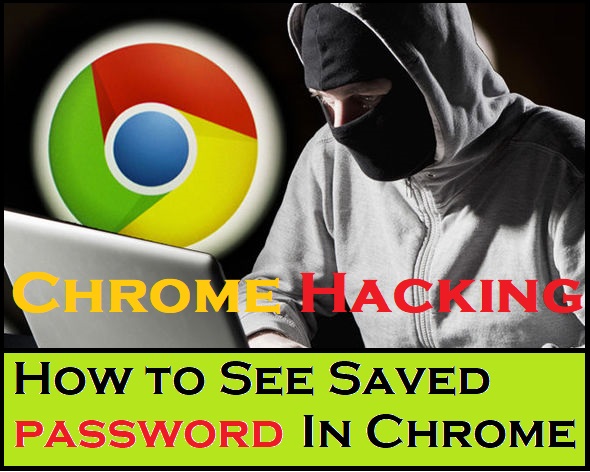
That JS consoles are lazy (and even deferred) has systematically been a pain point and a pain in the ass leading to eager deep cloning to ensure I can see what I actually have on hand at that point, especially in mutation-heavy code.

When I try to output something, my intent is to show the state of that thing at that point. > I think it would be more confusing if the console did not work like the rest of the language does. The language had been a thing for a decade at that point. It's also ahistorical revisionism "the console" was added very late into the history of the language, it and the entire console API were added by Firebug in the mid aughts. The primary purpose of `console.log` is and has always be to generate output from normal, non-interactive programs.Īnd it's completely wrong, `console.log` was absolutely intended as a logging method, as evidenced by its siblings `debug`, `info`, `warn` and `error`, pretty much like every logging API out there. > It's more that the way that the console reflects how the language works, and the console is not a log, it's a REPL (so console.output would have been a nicer name than console.log). I guess maybe if console.log() was restricted to strings, and something like console.logPresentation() was a separate function for printing objects, people would check the docs first and wouldn't be surprised. I think the only bad thing about console.log() is that this behavior is not taught to people as a core and important aspect of the function. Meanwhile, if you want to log a static snapshot of an object, all you need to do is to write console.log(cloneForLog(object)), where cloneForLog() is a function you wrote that does whatever copying is appropriate in your situation.
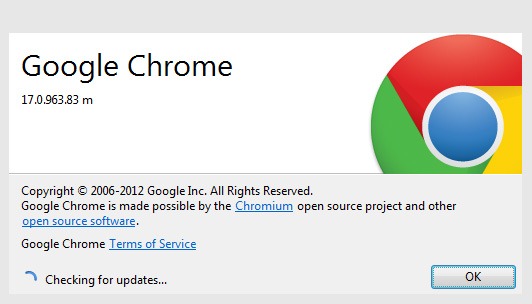
(Also, all these copies would not be garbage-collected until you cleared the console.)Īn universal deep copy is impractical to implement (notice how nobody seems to ever implement it, at all, in any programming language), and having console.log() do one would be an incredibly powerful and unpredictable footgun. An unlucky console.log() could easily copy half of your heap into the console, and god help you if you logged a DOM element. if two random objects A and B both have a pointer to the same object C, the copied A' and B' better both point at the same C').
#CONSOLE GOOGLE CHROME HACK FULL#
To log a static snapshot with equivalent interactive expansion capability, console.log() would have to do a general deep copy of your object - it would have to walk every pointer in the object and replace the pointee with its own recursive full deep copy, making sure to detect and handle cycles well, and keeping track of every replacement to ensure referential integrity (e.g. This is fast to do, and most of the time, it's exactly what you want. When you console.log() an object, it just stores a pointer to the object, and decorates it with an interactive label containing some text, so that it looks nice. The only thing that breaks the model is the transpilation and minification, without sourcemaps.įWIW, it's not like it could work in any other way. Print debugging is invaluable, but I can't help but think there's something of Smalltalk or Lisp in how you can mess with your app within a sandbox through the inspector, at runtime.
#CONSOLE GOOGLE CHROME HACK CODE#
You can get quite close to that after you've bundled your code and deployed it to a server, so long as you've got good source maps going on. I can't remember the last time I've used a console.log over setting a breakpoint in the debugger and fucking with the application state at that point to understand an issue. The console will reflect on the entire scope and annotate the code with their runtime values, the same as you get when working inside a JetBrains IDE.īut it's JS, so you can tweak it without committing it to disk and so you get some form of REPL driven development. You can hit Ctrl+Shift+P in the inspector and use the same kind of fuzzy file matcher you have in Sublime Text or VS Code, and from there you can set breakpoints, modify the code in memory, and so on. In a local build you basically have a 1:1 mapping of source code to deployed code and the debugger here basically becomes your IDE. The best trick for actual debugging is to leverage your browser console.
